How to Use Google Text-to-Speech API in JavaScript: A Comprehensive Guide
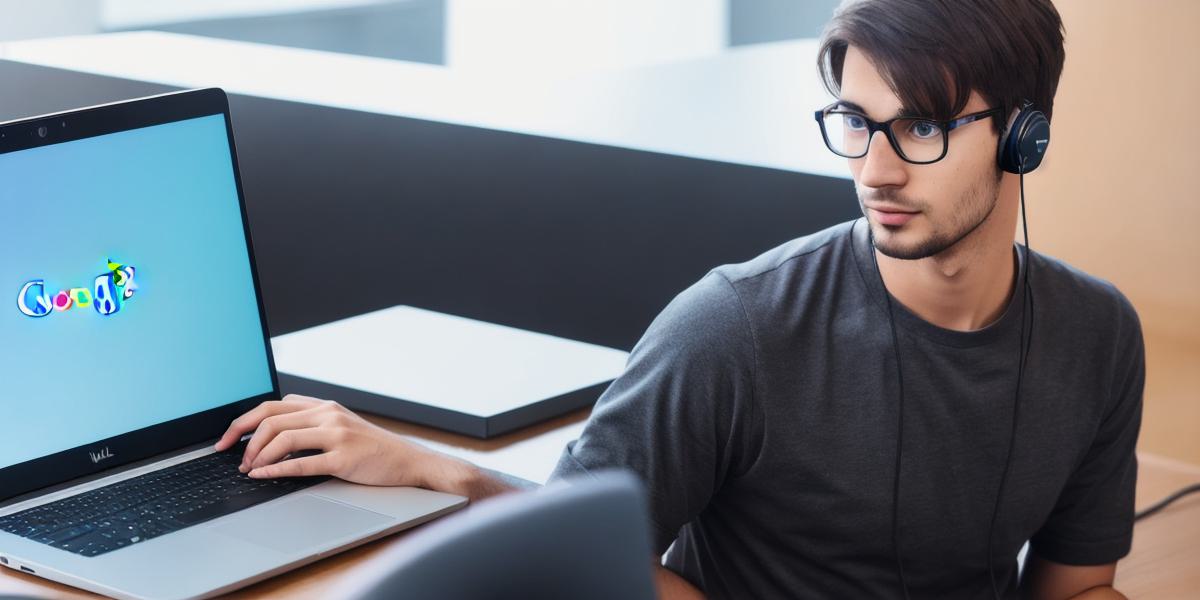
Introduction:
In today’s fast-paced world, AI developers are constantly seeking ways to optimize their workflow and make their applications more efficient. One such way is by utilizing the power of text-to-speech technology. Google’s Text-to-Speech API in JavaScript offers a simple and intuitive solution for developers looking to add voice functionality to their applications. In this comprehensive guide, we will explore everything you need to know about using the Google Text-to-Speech API in JavaScript, including how it works, its features, and best practices for implementation.
Using the Google Text-to-Speech API:
The Google Text-to-Speech API allows developers to convert written text into spoken words using a wide range of voices and languages. To use the API, you will first need to obtain an API key from the Google Cloud Console. Once you have your API key, you can begin by installing the required dependencies in your JavaScript project. These include the ‘web-speech-api’ library and the ‘googleapis’ package.
Next, you will need to import these libraries into your JavaScript file and create a new instance of the TextSynthesis object. This object is responsible for synthesizing spoken words from text input. You can then use the speak method on this object to output the spoken words to the user. For example:
const speech new webkitSpeechSynthesis();
speech.lang 'en-US';
function speakText(text) {
const utterance new SpeechUtterance(text);
speech.speak(utterance);
}
// Example usage:
speakText('Hello, world!');
Features of the Google Text-to-Speech API:
The Google Text-to-Speech API offers a wide range of features to help developers customize the voice and speech experience in their applications. These include:
- Customizable voices: With over 700 different voices available, developers can choose the perfect voice for their application.
- Multilingual support: The API supports more than 90 different languages, allowing developers to create applications that are accessible to users from all around the world.
- Pitch, rate, and volume control: Developers can adjust the pitch, rate, and volume of the spoken words to create a unique speech experience for their users.
- Markup support: The API supports several markup formats, including HTML, XML, and plain text, making it easy to integrate voice functionality into existing web applications.
Best Practices for Implementing the Google Text-to-Speech API in JavaScript:
To ensure that your implementation of the Google Text-to-Speech API is as efficient and effective as possible, here are a few best practices to follow:
- Use asynchronous programming: Since the API requires an internet connection to function, it’s important to use asynchronous programming techniques to avoid blocking the user interface.
- Cache API responses: To improve performance, consider caching API responses in memory or on disk to reduce the number of requests needed to access the same data.
- Use error handling: The API can throw errors if an invalid request is made or if there is a network issue. Be sure to handle these errors gracefully in your application.
- Optimize audio output: To ensure that the spoken words are clear and easy to understand, consider optimizing the audio output by adjusting the pitch, rate, and volume settings.
Conclusion:
In conclusion, the Google Text-to-Speech API in JavaScript is a powerful tool for developers looking to add voice functionality to their applications. With its wide range of features and customizable voices, this API can help make your applications more accessible and engaging for users from all around the world.