Is Python a Functional Language? A Deep Dive into Its Capabilities
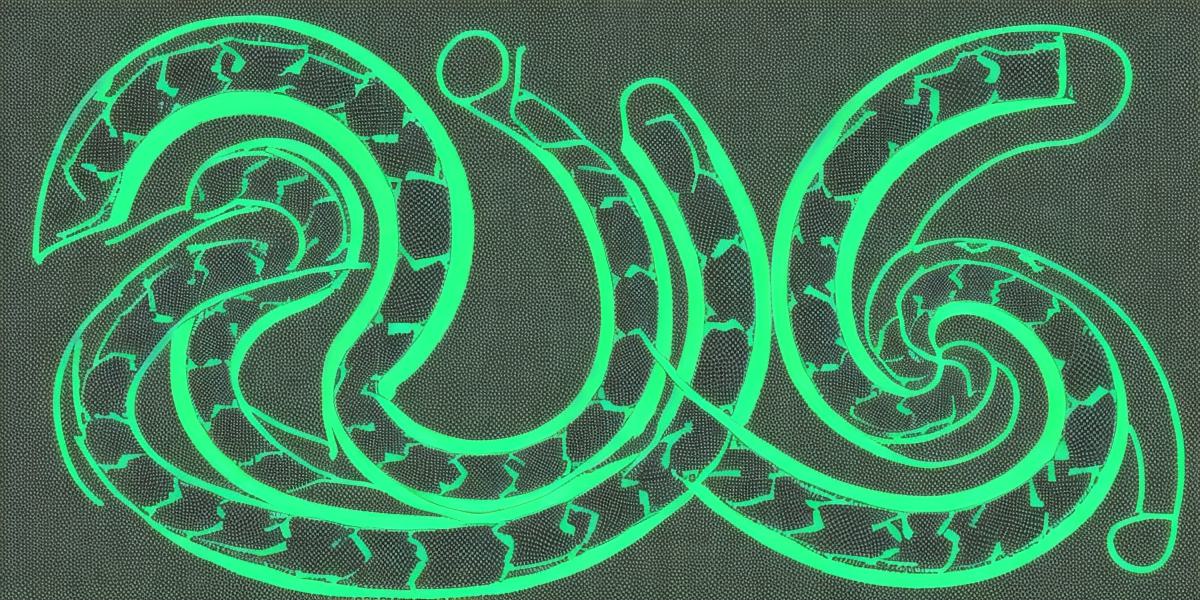
Python is one of the most popular programming languages out there, and for good reason. It’s easy to learn, has a vast library of modules, and is versatile enough to be used for web development, machine learning, data analysis, and much more. But is Python truly functional? In this article, we will explore the functional side of Python and see if it meets the criteria for being a functional language.
What is a Functional Language?
Before diving into Python’s capabilities, let’s first understand what a functional language is. A functional language is a programming paradigm that relies on mathematical functions to manipulate data and perform computations. In a functional language, the program’s output depends only on its input and does not have any side effects such as modifying a global state or interacting with an external system.
Functional Programming in Python
Python is often considered an imperative language since it supports both imperative and functional programming styles. However, functional programming can be achieved in Python by following some best practices and using certain libraries such as NumPy, Pandas, and Scikit-learn.
Pure Functions
A pure function is a function that does not have any side effects, meaning it only takes input and produces output without modifying any global state or interacting with an external system. In Python, pure functions can be defined using the lambda keyword, as shown in the example below:
def add(x, y):
return x + y
result add(2, 3)
print(result) Output: 5
In this example, the add
function takes two arguments and returns their sum. It does not have any side effects and can be considered a pure function.
Recursion
Recursion is a powerful technique in functional programming that allows functions to call themselves until a base case is reached. In Python, recursion can be used to solve problems such as Fibonacci sequence generation or sorting algorithms like QuickSort and MergeSort.
Here’s an example of a recursive function that generates the nth Fibonacci number in Python:
def fibonacci(n):
if n < 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(10)) Output: 55
In this example, the fibonacci
function takes an integer n
as input and returns its nth Fibonacci number using recursion.
Higher-Order Functions
Higher-order functions are functions that take other functions as input or output and can be composed with other functions to create new functions. In Python, higher-order functions can be used for sorting algorithms, filtering data, and mapping functions.
Here’s an example of a higher-order function that filters a list of integers in Python:
def filter_numbers(lst, predicate):
return [x for x in lst if predicate(x)]
print(filter_numbers([1, 2, 3, 4, 5], lambda x: x % 2 0)) Output: [2, 4]
In this example, the filter_numbers
function takes a list of integers lst
and a predicate function as input and returns a new list that contains only the elements for which the predicate function returns True
. The predicate function is a higher-order function that takes an integer as input and returns a Boolean value.
Immutability
Immutability is a key feature of functional programming languages, where data structures are not allowed to be modified once they are created. In Python, immutability can be achieved using tuples and sets, which cannot be modified after creation. However, lists can