How to Create Text-to-Speech in JavaScript: A Comprehensive Guide for AI Developers
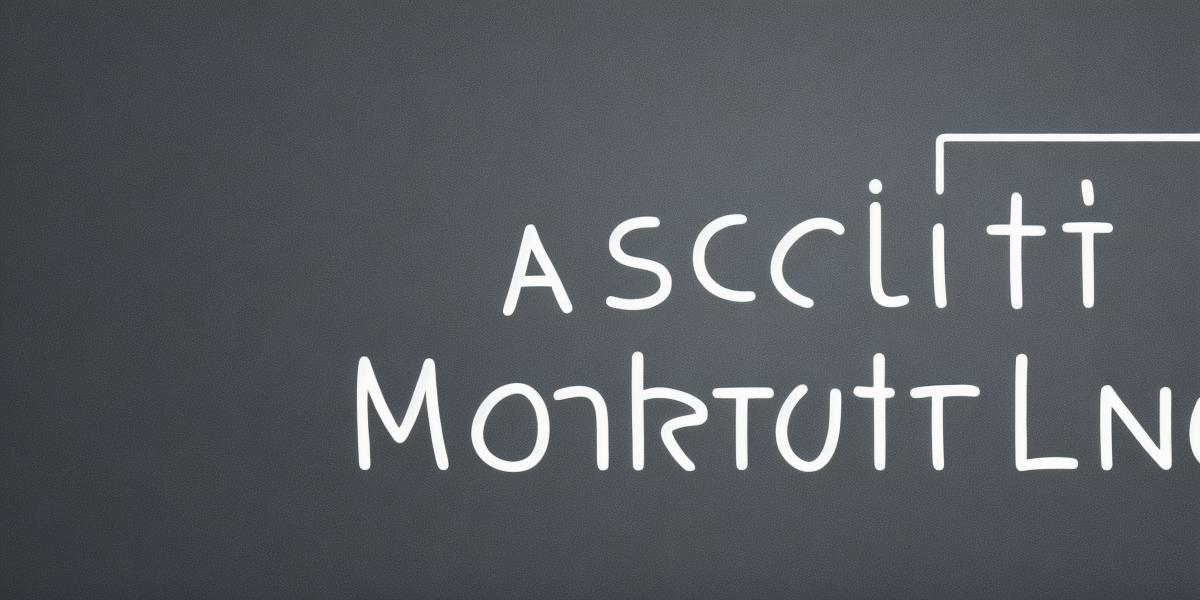
If you’re an AI developer looking to create text-to-speech capabilities in your applications, you’ve come to the right place. In this comprehensive guide, we’ll explore everything you need to know about creating text-to-speech in JavaScript.
First, let’s start with the basics. Text-to-speech (TTS) is a technology that converts written text into spoken words using synthesized voices. It has many practical applications, including e-learning, accessibility, and language translation.
Now, let’s dive into how to create TTS in JavaScript. There are several libraries available that can help you achieve this goal, such as Web Speech API, Festival.js, and Howler.js. In this guide, we will focus on the Web Speech API, which is a built-in JavaScript API that provides text-to-speech capabilities.
Here’s an example of how to use the Web Speech API to create TTS in JavaScript:
// Create a new speech synthesis object
const synth window.speechSynthesis;
// Set the voice and rate for the text-to-speech conversion
synth.voice 'en-US';
synth.rate 1.5;
// Define the text to be converted to speech
const text 'Hello, world!';
// Speak the text using the Web Speech API
synth.speak(text);
In this example, we first create a new speechSynthesis
object, which is used to interact with the TTS capabilities of the browser. We then set the voice and rate for the conversion. In this case, we’re using the English language (en-US) and speaking at a rate of 1.5 words per second.
Next, we define the text to be converted to speech. In this example, we’re simply saying "Hello, world!". Finally, we call the speak()
method on the synth
object to actually convert the text to speech and speak it out loud.
Now that you know how to create TTS in JavaScript using the Web Speech API, let’s take a closer look at some of the benefits and challenges of this technology.
Benefits
One of the main benefits of TTS is accessibility. By providing spoken words for visually impaired users, TTS can help them navigate web pages and other digital content more easily. Additionally, TTS can be useful for language translation, as it allows non-native speakers to hear the words being spoken in their native language.
Challenges
One of the main challenges of TTS is that it can sound robotic or unnatural. This is because TTS relies on synthesizing speech from individual sound units, which can result in a stilted and artificial-sounding voice. Additionally, TTS can be slow and resource-intensive, especially for long periods of time.
FAQs
- Is it possible to use TTS offline?
No, TTS requires an internet connection to access the necessary resources for synthesizing speech. However, you can cache some assets locally to improve performance. - How do I customize the voice and rate of TTS?
You can customize the voice and rate by setting the appropriate properties on thespeechSynthesis
object. For example, you can set the voice to a specific language or dialect, and adjust the rate to suit your needs. - What are some alternative libraries for creating TTS in JavaScript?
In addition to the Web Speech API, there are several other libraries available for creating TTS in JavaScript, including Festival.js and Howler.js. These libraries may offer different features and customization options than the Web Speech API.
Conclusion
Creating text-to-speech capabilities in your applications using JavaScript can be a powerful tool for improving accessibility and enhancing user experience. With the Web Speech API, you can easily convert written text into spoken words with just a few lines of code.