Mastering these 3 Essential Functional Skills for Success
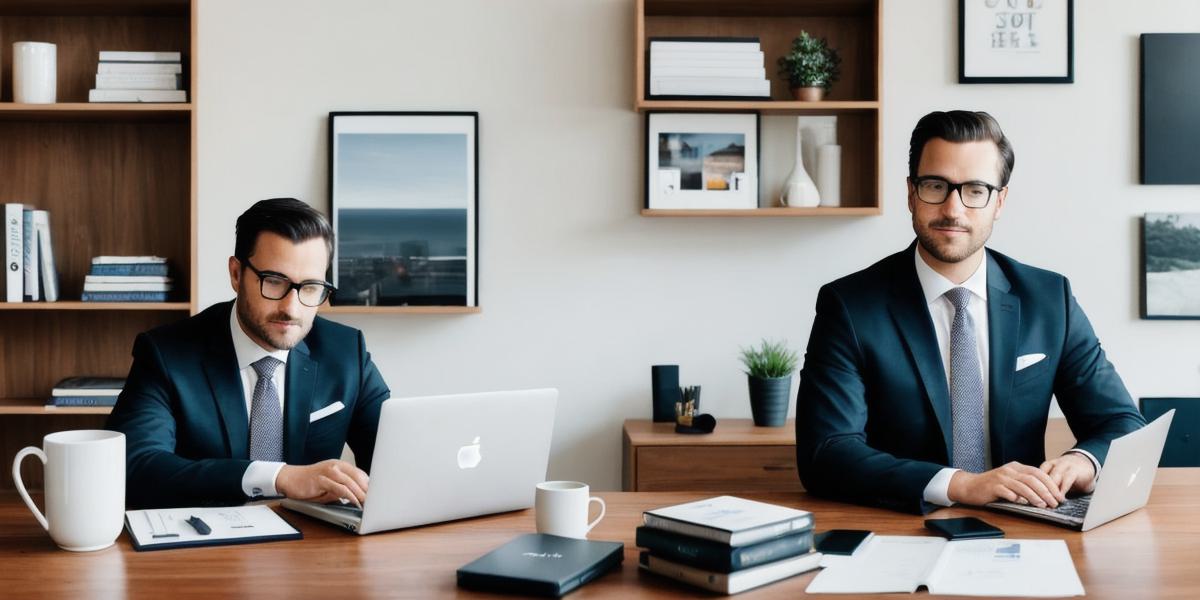
As a software developer, mastering functional programming skills can make all the difference in your success in the field. In this article, we will explore three essential functional programming skills that you should focus on to improve your abilities and take your career to the next level.
1. Purity vs Impurity
One of the fundamental principles of functional programming is the distinction between pure and impure functions. Pure functions are those that always produce the same output for a given input, while impure functions can have side effects such as changing the state of the program or accessing external resources.
To become a proficient functional programmer, you must understand this distinction and how it affects the design of your code. By writing pure functions whenever possible, you can create more predictable and reliable software that is easier to maintain and test.
For example, consider a function that calculates the sum of all even numbers in a list:
const sumEvenNumbers (numbers) > {
const sum numbers.reduce((total, num) > total + num, 0);
return sum;
};
In this case, sumEvenNumbers
is a pure function because it always produces the same output for a given input. However, if we modify it to read from an external file or make some other impure action, it would no longer be considered a pure function.
2. Higher-Order Functions
Higher-order functions are functions that take other functions as inputs or produce functions as outputs. They are a powerful tool in functional programming because they allow you to write more concise and expressive code.
Some common higher-order functions include map
, filter
, and reduce
. These functions can be used to transform data and perform complex operations on arrays, objects, and other data structures.
For example, suppose we have an array of numbers that we want to square:
const numbers [1, 2, 3, 4];
const squaredNumbers numbers.map(num > num * num);
console.log(squaredNumbers); // [1, 4, 9, 16]
In this case, map
is a higher-order function that takes an array of numbers and applies a transformation function (in this case, num > num * num
) to each element of the array. The result is a new array with the squared values of the original numbers.
3. Immutability
Immutability is another key concept in functional programming that refers to the principle of not changing the state of an object or data structure once it has been created. This can help prevent unintended side effects and make your code more predictable and easier to reason about.
To become proficient at immutability, you should learn how to create and manipulate immutable data structures such as arrays and objects using techniques like concatenation, mapping, and filtering.
For example, suppose we have an array of numbers that we want to sort in ascending order:
const numbers [4, 2, 1, 3];
const sortedNumbers numbers.slice().sort((a, b) > a - b);
console.log(sortedNumbers); // [1, 2, 3, 4]
In this case, we first create a copy of the original array using slice()
, which returns a new array containing all elements of the original array up to a specified index. We then sort the new array in ascending order using the sort()
method, which compares elements of the array and returns a sorted result.
FAQs
Q: What is the difference between pure and impure functions?
A: Pure functions always produce the same output for a given input, while impure functions can have side effects such as changing the state of the program or accessing external resources.
Q: What are some common higher-order functions in functional programming?
A: Some common higher-order functions include map
, filter
, and reduce
. These functions can be used to transform data and perform complex operations on arrays, objects, and other data structures.
Q: How do you create immutable data structures in functional programming?
A: You can create immutable data structures by using techniques like concatenation, mapping, and filtering, which allow you to manipulate the data without changing its state.